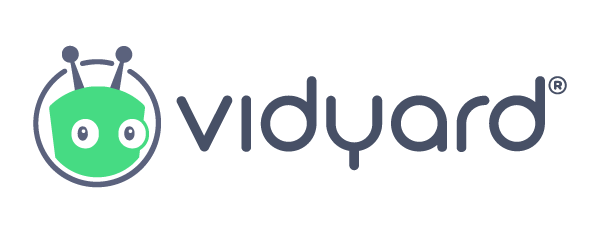
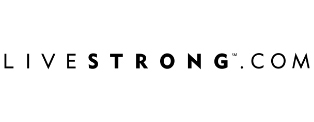
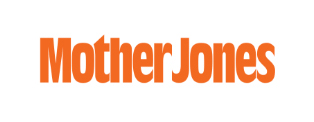
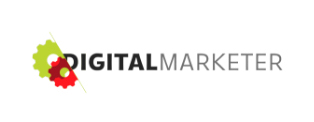
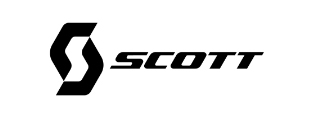
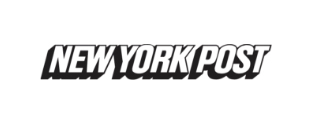
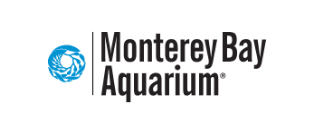
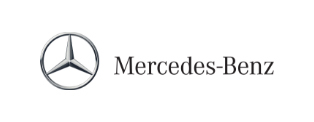
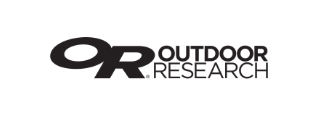
Flexible API Integrations Fit
for Every Application
JetSend’s on Stand-By, Ready to Implement with Any Application at Any Time.
1
curl --location --request POST
2
'https://app.jetsend.com/api/v1/transmission/email' \
3
--header 'accept: application/json' \
4
--header 'Authorization: Bearer [YOUR API KEY]' \
5
--header 'Content-Type: application/json' \
6
--data-raw '{
7
"email": {
8
"options": {
9
"description": "Testing Jetsend Email Transimission API",
10
"tracking_domain": "app-tracking@jetsend.xyz"
11
},
12
"recipients": [
13
{
14
"address": {
15
"email": "manjinder@maropost.com",
16
"name": "Manjinder Singh"
17
}
18
}
19
],
20
"content": {
21
"from": {
22
"name": "Jetsend Test Account",
23
"email": "test@jetsend.xyz"
24
},
25
"subject": "Welcome to Jetsend!",
26
"reply_to": "Jetsend Support <support@jetsend.xyz>",
27
"html": "<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='\''http://support.jetsend.com'\''>JetSend Support</a></p>this is just a test email."
28
}
29
}
30
}'
1
var request = require("request");
2
var options = {
3
method: 'POST',
4
'url': 'https://app.jetsend.com/api/v1/transmission/email',
5
headers: {
6
'accept': 'application/json',
7
'Authorization': 'Bearer [YOUR API KEY]',
8
'Content-Type': 'application/json'
9
},
10
body: JSON.stringify({"email":{"options":{"description":"Testing Jetsend Email Transimission API","tracking_domain":"app-tracking@jetsend.xyz"},"recipients":[{"address":{"email":"manjinder@maropost.com","name":"Manjinder Singh"}}],"content":{"from":{"name":"Jetsend Test Account","email":"test@jetsend.xyz"},"subject":"Welcome to Jetsend!","reply_to":"Jetsend Support <support@jetsend.xyz>","html":"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email."}}})
11
};
12
13
request(options, function (error, response) {
14
if (error) throw new Error(error);
15
console.log(response.body);
16
});
1
require 'uri'
2
require 'net/http'
3
4
url = URI("https://app.jetsend.com/api/v1/transmission/email")
5
6
https = Net::HTTP.new(url.host, url.port);
7
http.use_ssl = true
8
9
request = Net::HTTP::Post.new(url)
10
request["accept"] = "application/json"
11
request["Authorization"] = "Bearer [YOUR API KEY]"
12
request["Content-Type"] = "application/json"
13
request.body = "{\n \"email\": {\n \"options\": {\n \"description\": \"Testing Jetsend Email Transimission API\",\n \"tracking_domain\": \"app-tracking@jetsend.xyz\"\n },\n \"recipients\": [\n {\n \"address\": {\n \"email\": \"manjinder@maropost.com\",\n \"name\": \"Manjinder Singh\"\n }\n }\n ],\n \"content\": {\n \"from\": {\n \"name\": \"Jetsend Test Account\",\n \"email\": \"test@jetsend.xyz\"\n },\n \"subject\": \"Welcome to Jetsend!\",\n \"reply_to\": \"Jetsend Support <support@jetsend.xyz>\",\n \"html\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email.\"\n }\n }\n}"
14
15
response = https.request(request)
16
puts response.read_body
1
import requests
2
3
url = "https://app.jetsend.com/api/v1/transmission/email"
4
5
payload = "{\n \"email\": {\n \"options\": {\n \"description\": \"Testing Jetsend Email Transimission API\",\n \"tracking_domain\": \"app-tracking@jetsend.xyz\"\n },\n \"recipients\": [\n {\n \"address\": {\n \"email\": \"manjinder@maropost.com\",\n \"name\": \"Manjinder Singh\"\n }\n }\n ],\n \"content\": {\n \"from\": {\n \"name\": \"Jetsend Test Account\",\n \"email\": \"test@jetsend.xyz\"\n },\n \"subject\": \"Welcome to Jetsend!\",\n \"reply_to\": \"Jetsend Support <support@jetsend.xyz>\",\n \"html\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email.\"\n }\n }\n}"
6
7
headers = {
8
'accept': 'application/json',
9
'Authorization': 'Bearer [YOUR API KEY],
10
'Content-Type': 'application/json'
11
}
12
13
response = requests.request("POST", url, headers=headers, data = payload)
14
15
print(response.text.encode('utf8'))
1
package main
2
3
import (
4
"fmt"
5
"strings"
6
"net/http"
7
"io/ioutil"
8
)
9
10
func main() {
11
url := "https://app.jetsend.com/api/v1/transmission/email"
12
method := "POST"
13
14
payload := strings.NewReader("{\n \"email\": {\n \"options\": {\n \"description\": \"Testing Jetsend Email Transimission API\",\n \"tracking_domain\": \"app-tracking@jetsend.xyz\"\n },\n \"recipients\": [\n {\n \"address\": {\n \"email\": \"manjinder@maropost.com\",\n \"name\": \"Manjinder Singh\"\n }\n }\n ],\n \"content\": {\n \"from\": {\n \"name\": \"Jetsend Test Account\",\n \"email\": \"test@jetsend.xyz\"\n },\n \"subject\": \"Welcome to Jetsend!\",\n \"reply_to\": \"Jetsend Support <support@jetsend.xyz>\",\n \"html\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email.\"\n }\n }\n}")
15
16
client := &http.Client {
17
}
18
req, err := http.NewRequest(method, url, payload)
19
20
if err != nil {
21
fmt.Println(err)
22
}
23
24
req.Header.Add("accept", "application/json")
25
req.Header.Add("Authorization", "Bearer [YOUR API KEY]")
26
req.Header.Add("Content-Type", "application/json")
27
28
res, err := client.Do(req)
29
defer res.Body.Close()
30
body, err := ioutil.ReadAll(res.Body)
31
32
fmt.Println(string(body))
33
}
1
<?php
2
3
$request = new HttpRequest();
4
$request->setUrl('https://staging.jetsend.com/api/v1/email');
5
$request->setMethod(HTTP_METH_POST);
6
7
$request->setHeaders(array(
8
'content-type' => 'application/json',
9
'accept' => 'application/json',
10
'authorization' => '[YOUR_API_KEY]'
11
));
12
13
$request->setBody('{
14
"from_address": "[FROM_EMAIL_ADDRESS]",
15
"to_address": "[RECIPIENT_EMAIL_ADDRESS]",
16
"subject": "Welcome to Jetsend!",
17
"tags": ["new-user","alert"],
18
"tracking_domain": "[YOUR_VERIFIED_TRACKING_DOMAIN]",
19
"body": "<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href=\"http://support.jetsend.com\">JetSend Support</a></p>this is just a test email to test clicks and opens"
20
}
21
');
22
23
try {
24
$response = $request->send();
25
echo $response->getBody();
26
} catch (HttpException $ex) {
27
echo $ex;
28
}
1
var data =
2
JSON.stringify({"email":{"options":{"description":"Testing Jetsend Email Transimission API","tracking_domain":"app-tracking@jetsend.xyz"},"recipients":[{"address":{"email":"manjinder@maropost.com","name":"Manjinder Singh"}}],"content":{"from":{"name":"Jetsend Test Account","email":"test@jetsend.xyz"},"subject":"Welcome to Jetsend!","reply_to":"Jetsend Support <support@jetsend.xyz>","html":"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email."}}});
3
4
var xhr = new XMLHttpRequest();
5
xhr.withCredentials = true;
6
7
xhr.addEventListener("readystatechange", function() {
8
if(this.readyState === 4) {
9
console.log(this.responseText);
10
}
11
});
12
13
xhr.open("POST",
14
"https://app.jetsend.com/api/v1/transmission/email");
15
xhr.setRequestHeader("accept", "application/json");
16
xhr.setRequestHeader("Authorization", "Bearer [YOUR API KEY]");
17
xhr.setRequestHeader("Content-Type", "application/json");
18
19
xhr.send(data);
1
var client = new RestClient("https://staging.jetsend.com/api/v1/email");
2
var request = new RestRequest(Method.POST);
3
request.AddHeader("content-type", "application/json");
4
request.AddHeader("accept", "application/json");
5
request.AddHeader("authorization", "[YOUR_API_KEY]");
6
request.AddParameter("application/json", "{\n\t\"from_address\": \"[FROM_EMAIL_ADDRESS]\",\n\t\"to_address\": \"[RECIPIENT_EMAIL_ADDRESS]\",\n\t\"subject\": \"Welcome to Jetsend!\",\n\t\"tags\": [\"new-user\",\"alert\"],\n\t\"tracking_domain\": \"[YOUR_VERIFIED_TRACKING_DOMAIN]\",\n\t\"body\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens\"\n}\n", ParameterType.RequestBody);
7
IRestResponse response = client.Execute(request);
1
import Foundation
2
3
var semaphore = DispatchSemaphore (value: 0)
4
5
let parameters = "{\n \"email\": {\n \"options\": {\n \"description\": \"Testing Jetsend Email Transimission API\",\n \"tracking_domain\": \"app-tracking@jetsend.xyz\"\n },\n \"recipients\": [\n {\n \"address\": {\n \"email\": \"manjinder@maropost.com\",\n \"name\": \"Manjinder Singh\"\n }\n }\n ],\n \"content\": {\n \"from\": {\n \"name\": \"Jetsend Test Account\",\n \"email\": \"test@jetsend.xyz\"\n },\n \"subject\": \"Welcome to Jetsend!\",\n \"reply_to\": \"Jetsend Support <support@jetsend.xyz>\",\n \"html\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email.\"\n }\n }\n}"
6
let postData = parameters.data(using: .utf8)
7
8
var request = URLRequest(url: URL(string:
9
"https://app.jetsend.com/api/v1/transmission/email")!,timeoutInterval: Double.infinity)
10
request.addValue("application/json", forHTTPHeaderField: "accept")
11
request.addValue("Bearer [YOUR API KEY]", forHTTPHeaderField: "Authorization")
12
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
13
14
request.httpMethod = "POST"
15
request.httpBody = postData
16
17
let task = URLSession.shared.dataTask(with: request) { data, response, error in
18
guard let data = data else {
19
print(String(describing: error))
20
return
21
}
22
print(String(data: data, encoding: .utf8)!)
23
semaphore.signal()
24
}
25
26
task.resume()
27
semaphore.wait()
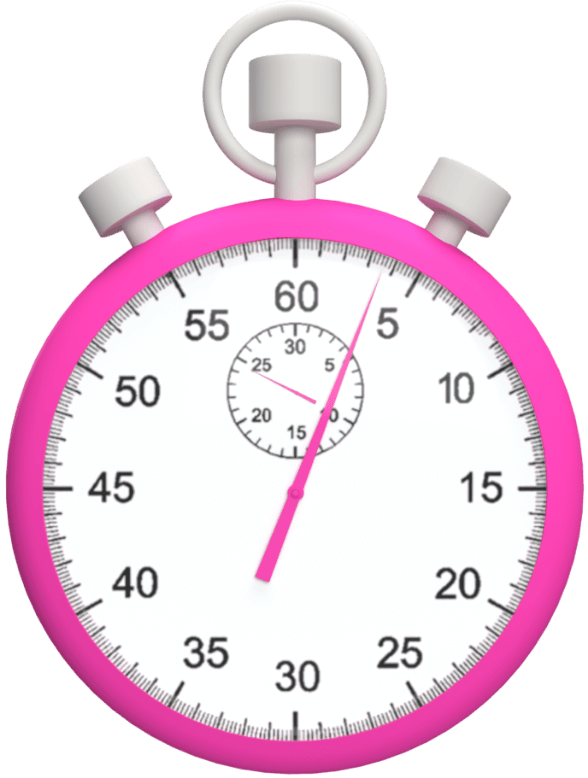
Timing is Everything!
JetSend follows the ‘5 seconds or less’ rule! Send speed is important, 70% of customers and users expect transactional emails to be delivered within 5 seconds of being triggered.